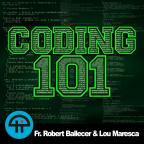
May 15th 2014
Coding 101 17
Python: Tuples
We review outputting lists, go deeper with sorting, then it's tuples time!
Welcome to Coding 101 - It's the TWiT show that gives YOU the knowledge to live in the wonderful world of the programmer. This week we are introducing our newest module, Python with Code Warrior Dale Chase!
To see all the code used in today's episode, go to Our Github Repository for Module 2
Printing Everything within a List:
The way we've been printing lists is to use a "for loop" to print everything within the list:
podcasters = ['Leo','Lisa','Padre', 'Snubs', 'Bryan', 'OMGchad']
for entries in range (0,6):
- print podcasters[entries]
- This will print each individual entry on its own line.
If we JUST wanted to know the contents of the list:
mylist = [142, 512, 'dog', 'cat', 'bird', 123]
print mylist
- This will print EVERYTHING that is in the list "mylist" - including the comas, spaces and brackets
Sorting the List
- We've been using "destructive sorting" with the "list.sort()" function
- When we use "sort()" on a list, we sort "IN PLACE" which destroys the original list
SortMe = [1, 23, 6, 4, 99]
for entries in range (0,5):
- print SortMe[entries]
raw_input ("Press Enter")
SortMe.sort();
for entries in range (0,5):
- print SortMe[entries]
NON-DESTRUCTIVE SORTING
- We can do a non-destructive sorting of a list.
- It keep the original list while sorting the data.
podcasters = ['Leo','Lisa','Padre', 'Snubs', 'Bryan', 'OMGchad', 1234]
print podcasters
print "Woot, let's sort!"
sortedpodcasters = sorted(podcasters)
raw_input("Press Enter to Continue")
print podcasters
print sortedpodcasters
- We can ALSO sort WITHOUT creating a new list
podcasters = ['Leo','Lisa','Padre', 'Snubs', 'Bryan', 'OMGchad', 1234]
print sorted(podcasters)
Tuples
- Tuples work like lists, but they are immutable. They cannot be changed.
- They use parentheses instead of brackets
- You can have a list of tuples.
twit_tuples = [
('Padre', 'TWiET', 72),
('Snubs', 'C101', 22),
('Bryan', 'KnowHow', 6),
]
print twit_tuples
Sorting Tuples
twit_tuples = [
('Padre', 'TWiET', 72),
('Snubs', 'C101', 22),
('Bryan', 'KnowHow', 6),
]
print sorted(twit_tuples, key=lambda twit: twit[2])
Get in Touch With Us!
- Subscribe and get Coding 101 automatically at https://twit.tv/shows/coding-101.
- Follow PadreSJ and Snubs on Twitter
Bandwidth for Coding 101 is provided by CacheFly.