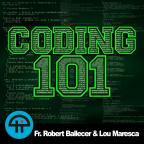
May 1st 2014
Coding 101 15
Python - Sort and the YouTube API
Sorting is one of the most useful programming functions you can learn. We not only teach you to sort, but we introduce you to the YouTube API as well.
Welcome to Coding 101 - It's the TWiT show that gives YOU the knowledge to live in the wonderful world of the programmer. This week we are introducing our newest module, Python with Code Warrior Dale Chase!
To see all the code used in today's episode, go to Our Github Repository for Module 2
Please Note: The episode code will NOT work unless you have your own YouTube API Key. The procedure for applying for your own YouTube API key can be found here.
Let's Learn about Sorting!
Let's say I have the following list of numbers: (1, 23, 6, 4, 99) Now I want to put that list into ascending order. I need to "sort" the data into an order that will be useful to me.
I could write a function that:
- Puts all the values into a list (rawlist)
- Creates a NEW list (sortlist) into which I will place those values as they are sorted
- Add the first value of (rawlist) into the first position of (sortlist)
- Compare each successive value of "rawlist" to the values that are already in (sortlist) and reorder (sortlist) accordingly
Here's how it would work:
- "1" is moved from (rawlist) to (sortlist)
- "23" from (rawlist) is compared to "1" in (sortlist). It is GREATER than "1" so it is added to (sortlist) AFTER "1"
- "6" from (rawlist) is compared to "1" in (sortlist). It is GREATER than "1" so it is then compared to "23" in (sortlist). It is LESSER than "23", so "6" is coppied into the position after "1" in (sortlist) and "23" is moved to the position after "6" in (sortlist)
- "4" from (rawlist) is compared against "1" in (sortlist). It is greater than "1", so it is compared against "6" in (sortlist). It is LESSER than "6", so "4" is coppied into the position after "1" in (sortlist), "6" is coppied into the position after "4" in (sortlist), and "23" is coppied into the position after "6" in (sortlist)
- "99" (rawlist) is compared against "1"(sortlist). It is greater than "1", so it is compared against "4" (sortlist). It is greater than "4", so it is compared against "6" (sortlist). It is greater than "6", so it is compared against "23" (sortlist). It is greater than, "23", so it is added to the position after "23" (sortlist)"
Python has a built-in sorting method!
sort()
- The "sort()" method will sort a list of objects, much in the same way that we just demonstrated.
- But we don't need to know all the logic behind the method!
Usage:
SortMe = [1, 23, 6, 4, 99]
for entries in range (0,5):
print SortMe[entries]
raw_input ("Press Enter")
SortMe.sort();
for entries in range (0,5):
print SortMe[entries]
Get in Touch With Us!
- Subscribe and get Coding 101 automatically at https://twit.tv/shows/coding-101.
- Follow PadreSJ and Snubs on Twitter
Bandwidth for Coding 101 is provided by CacheFly.